Last post we talked about Eureka
. It is one of the key elements of Spring Cloud. Service center as a critical service, if it is a single point and having a failure then it is devastating. In a distributed system, the service center is the most important part and should be in a state that can provide services at any time. To maintain its availability, using clusters is a good solution. Eureka
achieves high availability deployment by registering with each other, so we only need to configure Eureke Server
with other available serviceUrl
to achieve high availability deployment.
Two-node service center
First let’s try to setup a two-node service center.
1. Create application-peer1.properties
Peer1
service center’s configuration. Pointing serviceUrl
to peer2
.
1 | spring.application.name=spring-cloud-eureka-server |
2. Create application-peer2.properties
Peer2
service center’s configuration. Pointing serviceUrl
to peer1
.
1 | spring.application.name=spring-cloud-eureka-server |
3. Host file
Add the following 2 lines to hosts
file
1 | 127.0.0.1 peer1 |
Start server
Run the following command
1 | # package jar file |
Visit http://localhost:8000/
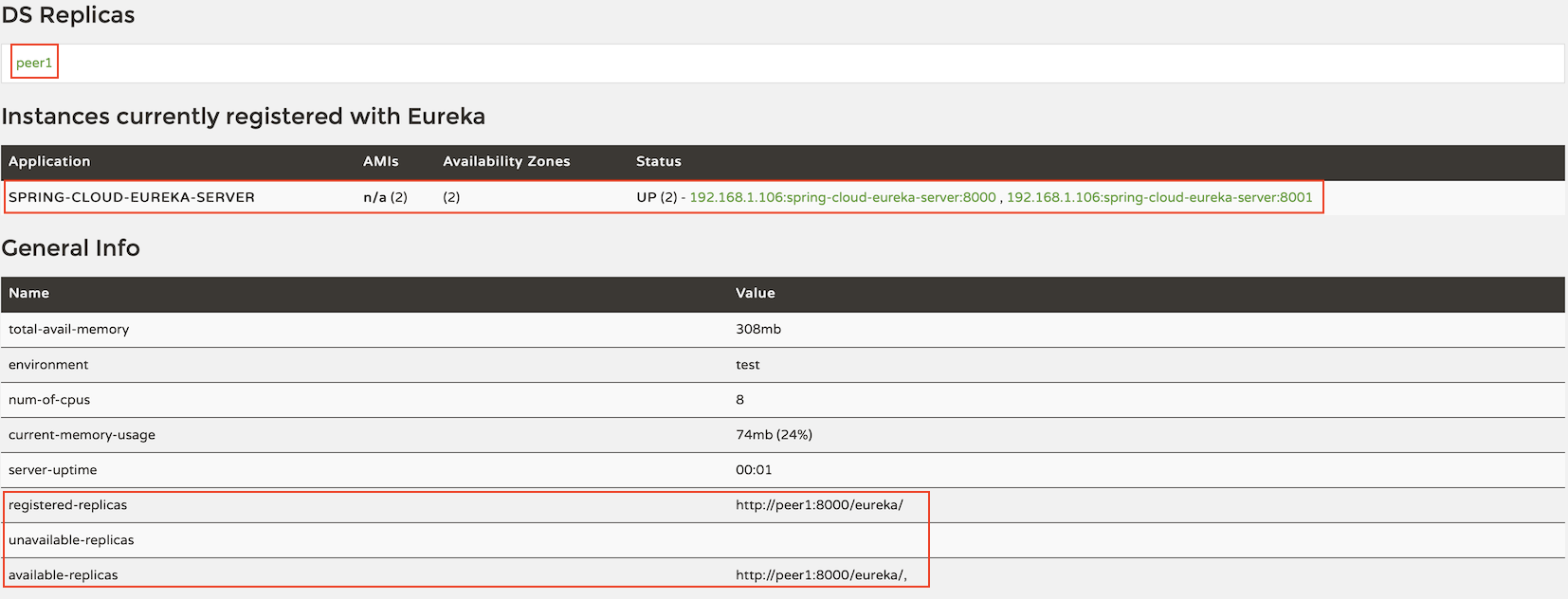
We can see that peer1
‘s service center DS Replicas already has the configuration information of peer2
, and peer2
appears in available-replicas. If we manually stopped peer2
, then peer2
would move to the unavailable-replicas column, indicating that peer2 was unavailable.
Use eureka cluster
In production, we may need three or more service centers to ensure the stability of the service. The configuration are actually the same: pointing the service centers to other service centers. Here I will only introduce the configuration of only three clusters. In fact, it is similar to the two-node service center. Each service center can point to the other two nodes.
application-peer1.properties
1 | spring.application.name=spring-cloud-eureka-server |
application-peer2.properties
1 | spring.application.name=spring-cloud-eureka-server |
application-peer3.properties
1 | spring.application.name=spring-cloud-eureka-server |
Start peer1
, peer2
, peer3
eureka server
1 | java -jar spring-cloud-eureka-0.0.1-SNAPSHOT.jar --spring.profiles.active=peer1 |
Visit http://localhost:8000/
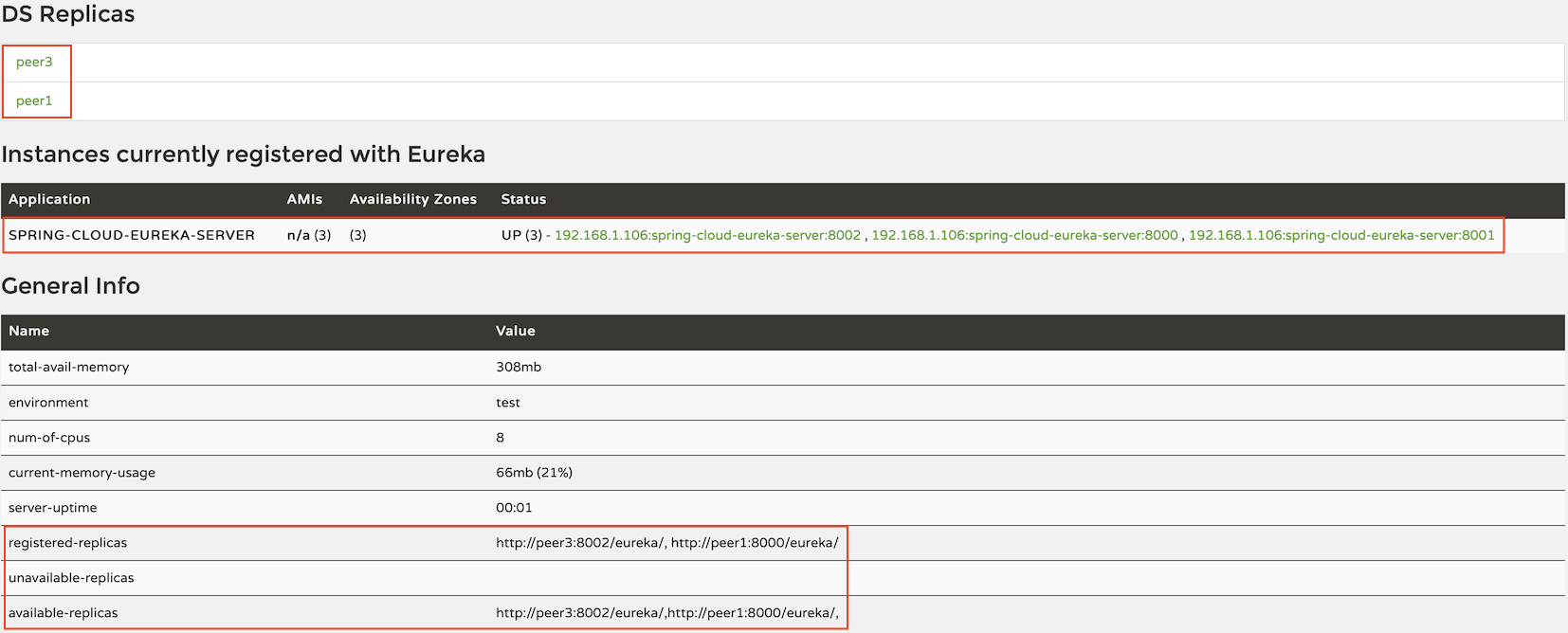
We can see peer2
and peer3
‘s information in peer1
Check out the source code here: Eureka cluster demo