Question
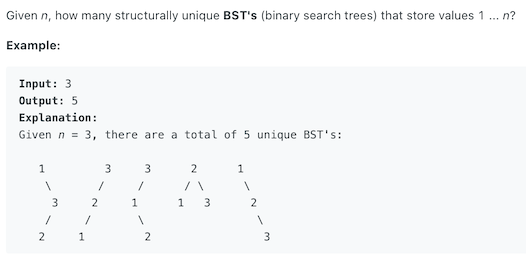
Similar to 95. Unique Binary Search Trees II. This time just need to count the number of unique binary search tree.
Similar Questions
- Medium - 95. Unique Binary Search Trees II
Solution
The following is the analysis for 95. Unique Binary Search Trees II:
Example:
1....n
Take1
as the root node,[]
as the left subtree, and all of[2 ... n]
as the right subtree.
Take2
as the root node,[1]
as the left subtree,[3 ... n]
as the right subtree.
Take3
as the root node,[1 2]
as the left subtree,[4 ... n]
as the right subtree. Then pair the left and right subtrees.
Take4
as the root node,[1 2 3]
as the left subtree,[5 ... n]
as the right subtree. Then pair the left and right subtrees.
…
Taken
as the root node,[1 ... n]
as the left subtree,[]
as the right subtree.
If there is only one number, then there is only 1 case, using that number as a tree. If it is [], it returns null.
For this problem, it will be simpler, we only need to return the number of trees. To find the number of subtrees of current roots, just multiply the number of left subtrees by the right subtree.
As there are many repeated calculations, we can save the results obtained during the recursion, and it can be taken directly when needed for the second time.
1 | // dynamic programming values, map n -> number of valid trees |