Question
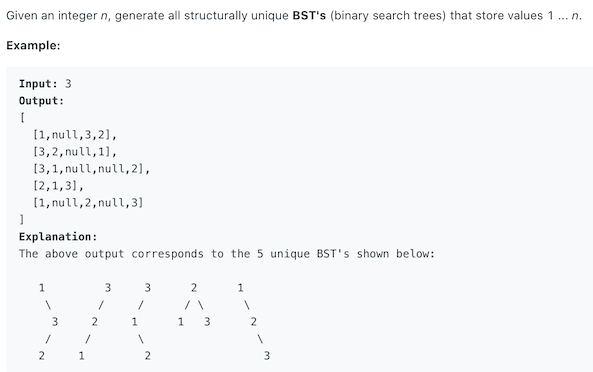
Given n, use these numbers 1 ... n to generate all possible binary search trees.
Similar Questions
- Medium - 96. Unique Binary Search Trees
- Medium - 241. Different Ways to Add Parentheses
Solution
One of the most important property of binary tree: all values of the left subtree are smaller than the root node, and all values of the right subtree are larger than the root node. We could use it to solve this problem
Example: 1....n
Take 1
as the root node, []
as the left subtree, and all of [2 ... n]
as the right subtree.
Take 2
as the root node, [1]
as the left subtree, [3 ... n]
as the right subtree.
Take 3
as the root node, [1 2]
as the left subtree, [4 ... n]
as the right subtree. Then pair the left and right subtrees.
Take 4
as the root node, [1 2 3]
as the left subtree, [5 ... n]
as the right subtree. Then pair the left and right subtrees.
…
Take n
as the root node, [1 ... n]
as the left subtree, []
as the right subtree.
If there is only one number, then there is only 1 case, using that number as a tree. If it is [], it returns null.
1 | public List<TreeNode> generateTrees(int n) { |