Question
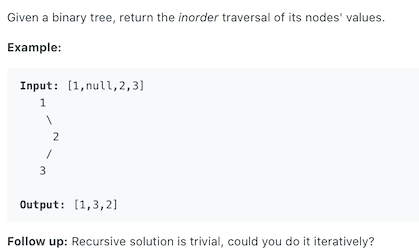
In-order traversal of a binary tree.
Similar Questions
- Medium - 98. Validate Binary Search Tree
- Medium - 144. Binary Tree Preorder Traversal
- Medium - 145. Binary Tree Postorder Traversal
- Medium - 173. Binary Search Tree Iterator
- Medium - 230. Kth Smallest Element in a BST
- Medium - 783. Minimum Distance Between BST Nodes
Solution - Recursive
Using recursion to traverse a binary tree. This is one of the standard approach.
1 | public List<Integer> inorderTraversal(TreeNode root) { |
Time complexity: O(n), traverse each node.
Space complexity: O(h), stack consumption, h is the height of the binary tree.
Solution - Stack
Use the stack to simulate recursion.
Let’s look at an example:
1 | 1 |
Code:
1 | List<Integer> result = new ArrayList<>(); |
Time complexity: O(n), traverse each node.
Space complexity: O(h), stack consumption, h is the height of the binary tree.