Question
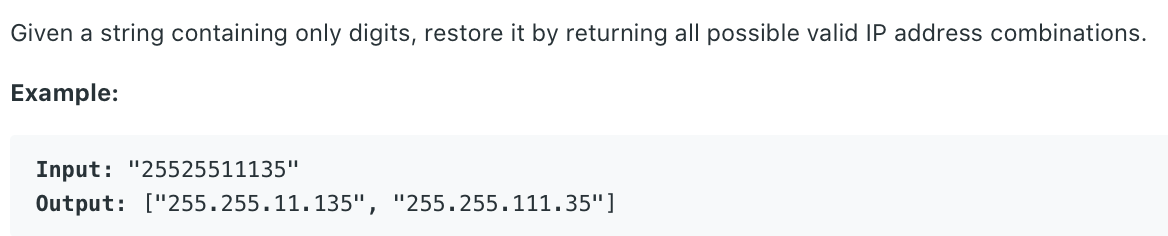
Give a string and output all possible IP addresses. Note that results like 01.1.001.1 which starts with 0 is an illegal string.
Solution - Brute Force
Because we know that the string needs to be divided into 4 parts, we directly use three loops to force the string into four parts, traverse all the divisions, and then choose a valid solution.
1 | public List<String> restoreIpAddresses(String s) { |
Solution - Backtracking
This question is actually dividing a string, and the number of divisions has been determined, which is 4 parts. So we can use the idea of backtracking directly. The first part may be 1 digit, and then enter the recursion. The first part may be 2 digits and then go into recursion. The first part might be 3 digits and then go into recursion.
1 | public List<String> restoreIpAddresses(String s) { |