Question
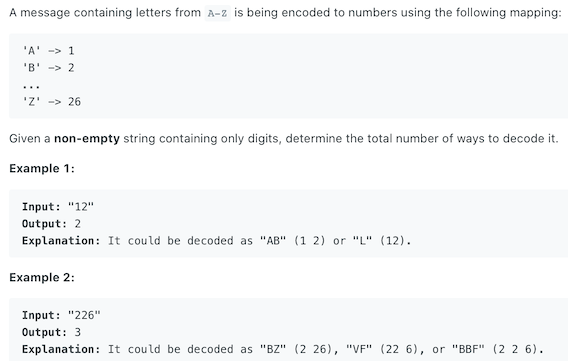
Each number corresponds to a letter. Given a string of numbers, need to know how many decoding methods. For example, 226 can have three decode ways, 2|2|6, 22|6, 2|26.
Similar Questions
- Hard - 639. Decode Ways II
Solution 1 - Recursion
It is easy to think of using recursion, turning big problems into smaller problems.
For example, 232232323232
.
For the first letter we have two divisions.
2|32232323232
and 23|2232323232
Therefore, if we know that the decoding result of the right part 32232323232
is ans1
and that of 2232323232
is ans2
, then the overall 232232323232
decoding result is ans1
+ ans2
.
If it is too hard to understand, think about this analogy.
If there are two roads from Melbourne to Brisbane, via Sydney and Canberra. There are 8 roads from Sydney to Brisbane, and 6 roads from Canberra to Brisbane. So there are 8 + 6 = 14 routes from Melbourne to Brisbane.
1 | public int numDecodings(String s) { |
Solution 2 - Recursion + Dynamic Programming
In solution 1, after calculating ans1
and then calculate ans2
, some already calculated results will be recalculated, so we can use the memoization technique to calculate a result and save it.
1 | public int numDecodings(String s) { |