Question
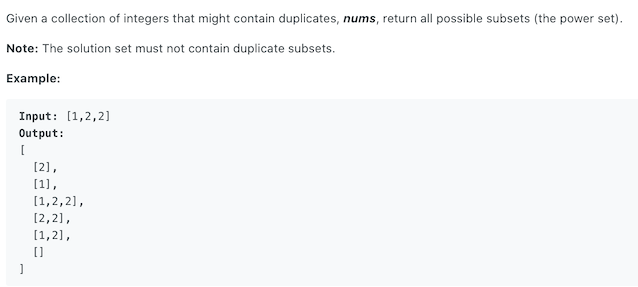
Return all subsets of a set of integers (may contain duplicates).
Similar Questions
- Medium - 78. Subsets
Solution
We can revise the solution of 78. Subsets. Let’s take a look at what would happen if we followed the idea of 78. Subsets directly. The previous idea was to add in first element, then add in second element to the subset formed previous. With n-th element, that is add n-th element to all previously formed subset
For example, the iteration process of nums[1, 2, 3].
1 | initialize empty list: [] |
But what happens if there are duplicate numbers?
E.g, nums[1, 2, 2]
1 | 1st iteration (nums[k] = 1):[[], [1]] |
We noticed that there are duplicate arrays.
How to avoid it? We can make a Hashset to store the results, everytime we have a new result, first sort the result, and try to add it to the set. This way we can avoid adding duplicates to the result set. Finally just need to convert the set to a list.
1 | // use set to avoid duplicate results |