Question
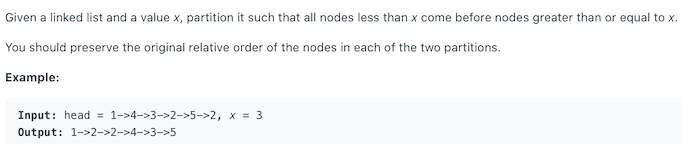
The question is essentially the partition of quick-sort. The linked list is divided into two parts, one part is less than the partition point x
, and the other part is greater than or equal to the partition point x
.
Solution
Looking at the quick-sort algorithm. In the quick-sort, we used two pointers for pivoting. One pointer indicates that the number before the pointer is less than the pivot point. The other pointer traverses the array.
1 | 1 4 3 2 5 2 x = 3 |
This question is just replacing array with linked list, and the question requires that the relative position of the numbers cannot be changed. So we must not use the exchange strategy, let alone the linked list exchange is more troublesome. In fact, we can just use the insert.
Similarly, a pointer is used to record the end of the linked list that is currently smaller than the pivot point, and another pointer is used to traverse the linked list. Each time we meet a number less than the pivot point, it is inserted into the end of the linked list and the end pointer is updated. We can use a dummy node to reduce the judgment of boundary conditions.
1 | // pivot node, first element that is >= x |
Time complexity: O(n).
Space complexity: O(1).