Question
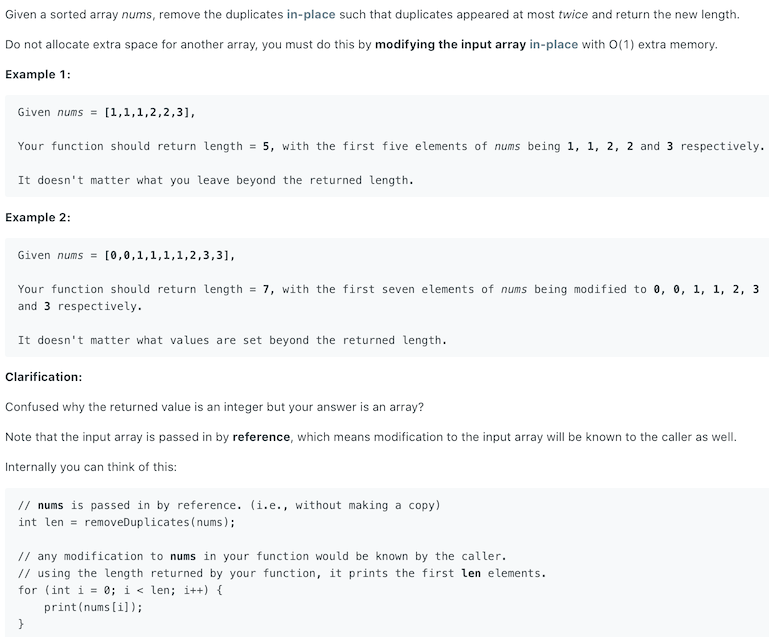
Remove duplicates from a sorted array in-place, such that duplicates appears at most twice.
Similar Questions
Solution
Make use of 2 pointers. Slow pointer
point to the element that satisfy the condition (appears at most twice). Fast pointer
always move forward and loop through the array. We can use a variable to record how many times the element of slow pointer
have occurred.
1 | int slow = 0; |