Question
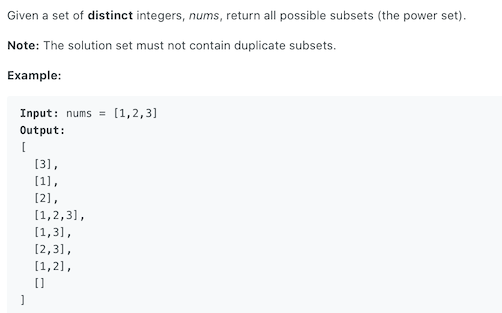
Return all subsets of a set of distinct integers.
Similar Questions
- Medium - 90. Subsets II
- Medium - 784. Letter Case Permutation
Solution - Iteration
First consider all subset of 1 element of the given array, then all subset of 2 elements of the array … and finally all subset of n elements of the array. To find all subset of k elements, just add nums[k]
to all subset of k-1 element.
For example, the iteration process of nums[1, 2, 3].
1 | initialize empty list: [] |
1 | // result list |
Other solutions: backtracking
Other solutions: bit manipulation