Question
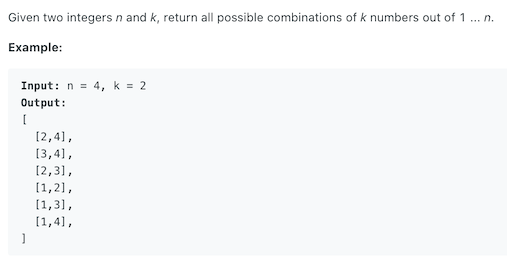
Given n
, k
, select k
numbers from {1, 2, 3 ... n}
, output all possible, and sort numbers from small to large, and each number can only be used once.
Similar Questions
- Medium - 39. Combination Sum
- Medium - 46. Permutations
Solution
Very classic backtracking problem. First select a number, then enter the recursion to continue to select, meet the conditions and add to the result, then back to the previous step and continue the recursion.
Look at the code directly, it is easy to understand.
1 | public List<List<Integer>> combine(int n, int k) { |
A for loop, add
, recurse
, delete
, is a classic backtracking template. Here is an optimization method. In the for loop, i
goes from start
to n
, but it is not necessary to go to n
. For example, n = 5
, k = 4
, tempList.size () == 1
, which means that we need (4-1 = 3)
numbers. If i = 4
, we will at most add 4 and 5 to tempList
. In this case, tempList.size()
is equal to 1 + 2 = 3
, which is less than 4, so i
does not need to be equal to 4, and it is sufficient for i
to loop to 3.
So the end condition of the for
loop can be changed to i <= n-(k-tempList.size()) + 1
, k-tempList.size()
represents the numbers we need. Because we got n
in the end, we need to add 1.
1 | public List<List<Integer>> combine(int n, int k) { |
Although only one line of code is changed, the speed is much faster.
There are other methods, such as recursion
, iteration
, dynamic programming
can be applied here, which I will be discuss in the future if have time.