Question
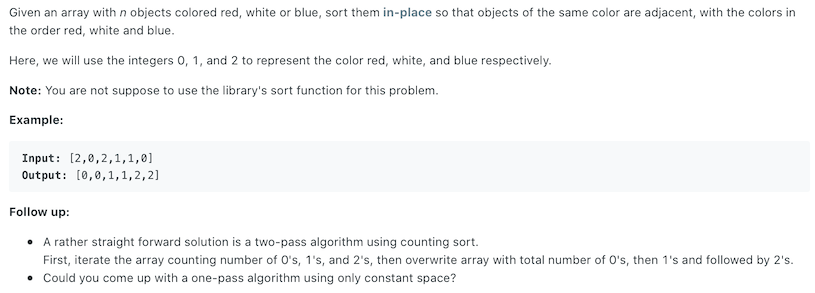
Give an array containing only 0, 1, and 2, sort the numbers from small to large.
Similar Questions
- Medium - 148. Sort List
- Medium - 324. Wiggle Sort II
Solution 1
A very straightforward method. Requires 2 iterations. During first iteration, count the number of occurrences of 0, the number of occurrences of 1, and the number of occurrences of 2. During second iteration, traverse the array and change the elements of the array to the corresponding values according to the number of occurrences. Of course we only need to record 0 and 1, and the rest is 2.
Time complexity O(n), space complexity O(1).
Solution 2
Solution 1 requires 2 iterations. Can we do better in only 1 iteration?
Suppose the array only have number 0 and 1 (e.g, 1 0 1 1 0
). We can use a pointer, zero_position
, meaning the positions before this pointer are 0
. Then use a pointer i
to traverse the array, find 0 and put 0 to the position pointed by zero_position
, and move zero_position
forward. Let Z
be zero_position
and see the traversal process below.
1 | 1 0 1 1 0 Initialize Z,i point to position 0,move forward i |
Now We have 3 numbers, so we can use two pointers, one is zero_position
. As before, all the positions before it are all 0. Another pointer, two_position
. All the positions after it are all 2.
1 | 0 1 0 2 1 2 2 2 |
1 | // elements before this are all '0' |
Time complexity O(n), space complexity O(1).