Question
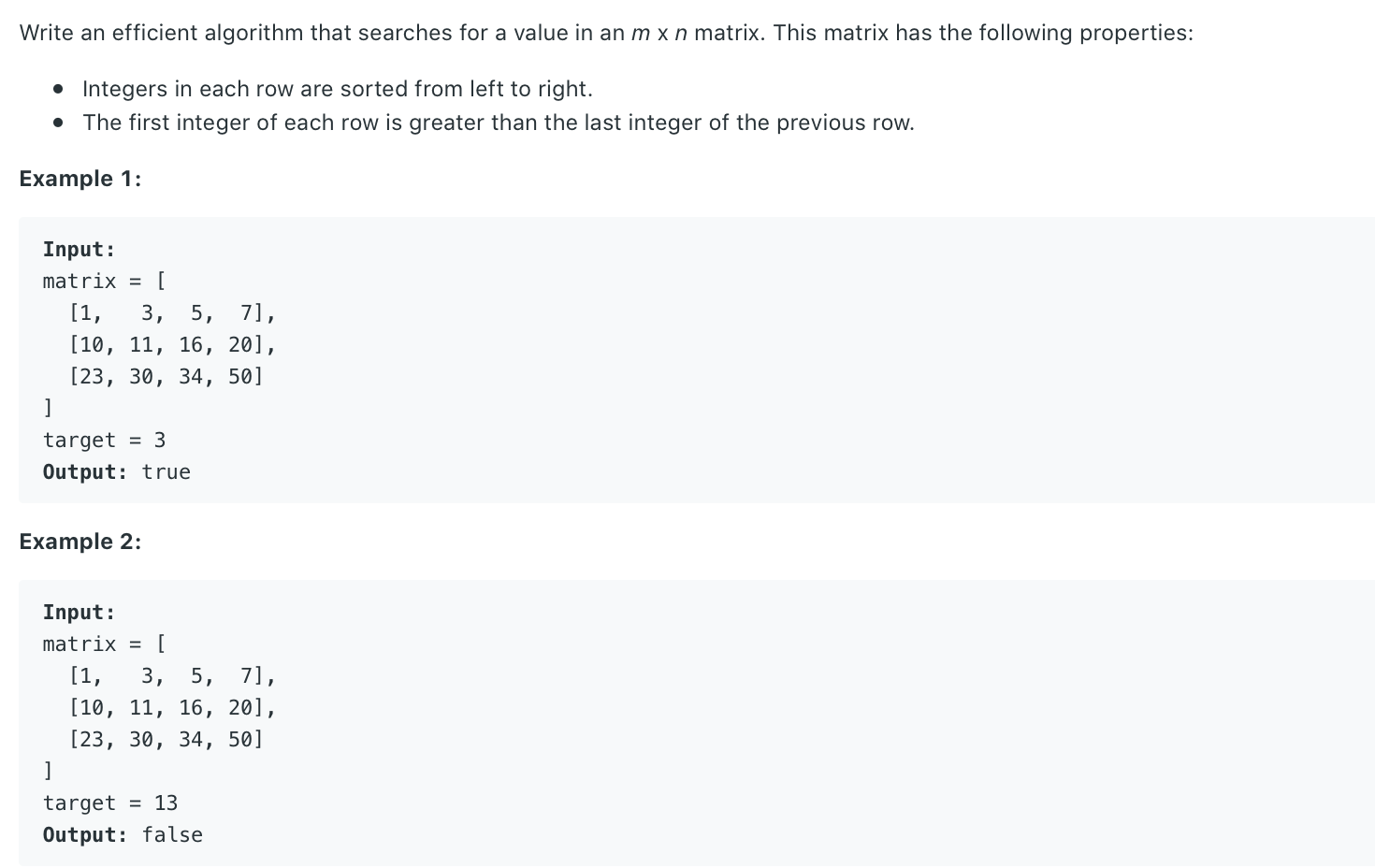
Search a value in an m x n matrix, determine if it exists.
Similar Questions
- Medium - 240. Search a 2D Matrix II
Solution
As the martix is ordered, there is no doubt that we need to use binary search. First find which row the target belongs to, then use binary search to determine. We just need to consider how to convert the index into the row and column of the matrix. To do so, we can use division and mod.
1 | // matrix is empty |