Question
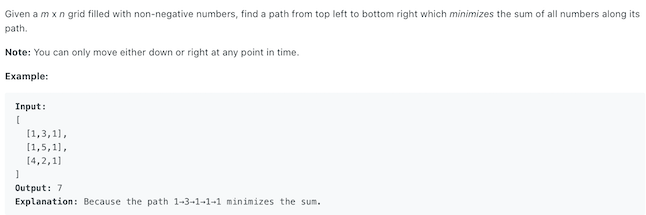
From top left to bottom right, find a path that minimize the sum along the path.
Similar Questions
- Medium - 62. Unique Paths
- Hard - 174. Dungeon Game
- Hard - 741. Cherry Pickup
Solution
Define a 2D array. Each element of the array represent the minimum sum starting from top left point to this point. For example, dp[i][j] = 5
means that starting from (0, 0)
to (i, j)
, the minimum sum is 5.
The value on the first row, it is only reachable from its left point. And first column is only reachable from its up point.
For other points, the minimum sum is the sum of itself and minimum value of the point up it and left to it. Thus we have our dynamic programming formula: dp[i][j] = grid[i][j] + Math.min(dp[i - 1][j], dp[i][j - 1])
. And we start to fill the dp array from second row.
1 | // result grid |