Question
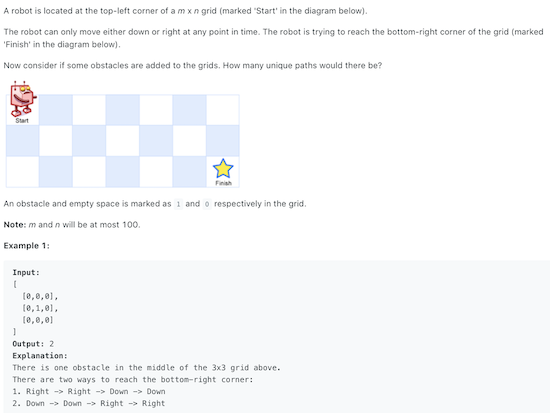
Similar to Question 62: Unique Paths. This time given a input array to mark obstacles.
Similar Questions
- Medium - 62: Unique Paths
- Hard - 980. Unique Paths III
Solution
The solution is similar to Question 62: Unique Paths. Define a 2D array result
. Each element of result
represent the unique path starting from this point to bottom-right point.
The point on the last row and last column can only have 1 unique path (because we can only move right and down). However, if we traverse from the last to the first (e.g, from right to left or bottom to top), if there are any obstacle on the route, then the rest on the last row and last column is 0, as this route is blocked.
For other points, the number of unique paths is determined by unique path of the point below it and right to it. There are 3 situations:
- If both bottom and right point is obstacle or the position itself is obstacle, set to 0
- If only one of bottom and right point is obstacle, set to right or bottom
- Otherwise,
result[i][j] = result[i + 1][j] + result[i][j + 1]
.
Finally we just need to get the value of result[0][0]
1 | // get number of columns and rows |