Question
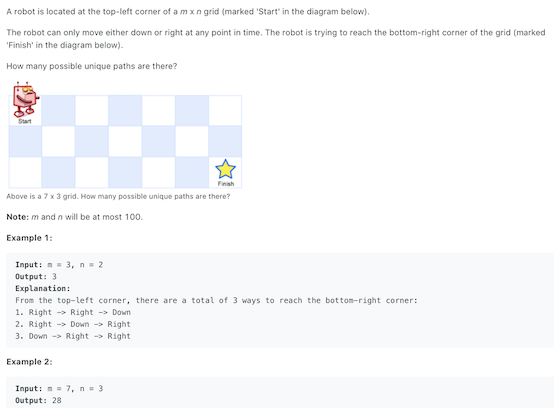
Count the number of paths from top-left corner to bottom-right corner. Can only move down or right.
Similar Questions
- Medium - 63: Unique Paths II
- Medium - 64. Minimum Path Sum
- Medium - 174. Dungeon Game
Solution
Use the idea of dynamic programming, we can define a 2D array. Each element of the array represent the unique path starting from this point to bottom-right point. For example, dp[i][j] = 5
means that starting from (i, j)
, there are 5 unique paths.
Let’s observe the problem. The first insight we can have is that the point on the last row and last column can only have 1 unique path (because we can only move right and down).
For other points, the number of unique paths is the sum of unique path of the point below it and right to it. Thus we have our dynamic programming formula: dp[i][j] = dp[i + 1][j] + dp[i][j + 1]
. And we start to fill the dp array from second last row.
1 | // dp array |
Time complexity O(n * m), space complexity O(n * m).