Question
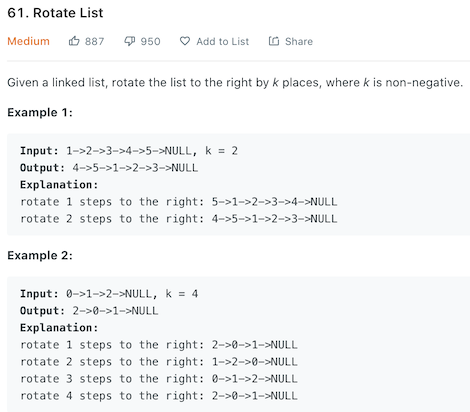
Move the last linked list node to the front and repeat the process k
times.
Similar Questions
- Easy - 189. Rotate Array
- Medium - 725. Split Linked List in Parts
Solution
Obviously we don’t really need to move nodes one by one. If the length of the linked list is len
and n = k % len
, we only need to move the last n
nodes to the front. We only need to find the pointer of the inverse n + 1
node and point it to null, and the pointer at the end to the head node. Finding the last n
nodes reminds me of 19. Remove Nth Node From End of List, using the fast and slow pointers.
1 | public static ListNode rotateRight(ListNode head, int k) { |
Time complexity O(n).
Space complexity O(1).