Question
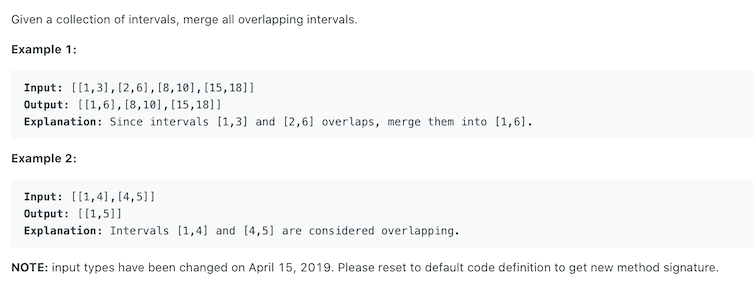
Merge the intervals that have overlaps.
Similar Questions
- Hard - 57. Insert Interval
- Medium - 495. Teemo Attacking
- Hard - 715. Range Module
- Medium - 763. Partition Labels
- Medium - 986. Interval List Intersections
Solution
Suppose given an array of size n
, then we assume that the first n-1
elements has been merged. What we have to solve now is the remaining one, how to add it to the n-1
elements that have been merged.
What we can do here is first sort the whole array by the left endpoint of the interval. Then loop the whole array, and adding in the new one with the last element of already merged intervals.
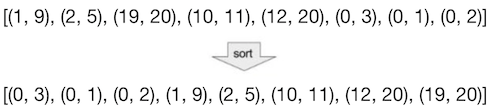
After sorting, we can simply compare the new interval with the last interval in the result set, see if they have overlap.
- Case 1: If the left endpoint of the new interval is greater than the right endpoint of the last interval of the result list, then just add the new interval directly.
For example:
Result list: (1, 6), (8, 12)
New interval: (15, 19)
New result list: (1, 6), (8, 12), (15, 19)
- Case 2: If the left endpoint of the new interval is not larger than the right endpoint of the last interval of the result list then only the right endpoint of the new interval and the right endpoint of last interval of the result list need to be determined.
Result list: (1, 6), (8, 12), (15, 19)
New interval: (17, 21)
New result list: (1, 6), (8, 12), (15, 21)
1 | public int[][] merge(int[][] intervals) { |