Question
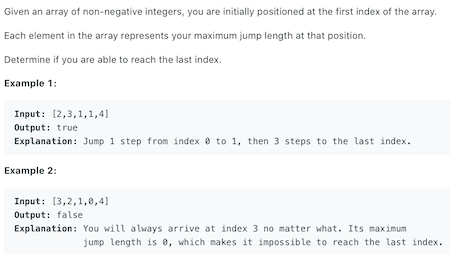
Jump from the 0th index of the array, the distance of the jump is less than or equal to the corresponding number in the array. Determine if can reach the last index.
Similar Questions
- Hard - 45. Jump Game II
Solution
We need to know from i-th
position, what is the furthest position it can reach. If any of the element has a furthest position that can reach end, then return true
. If reach is position that can’t be reached from previous elements, then return false
.
1 | if (nums.length == 1) { |