Question
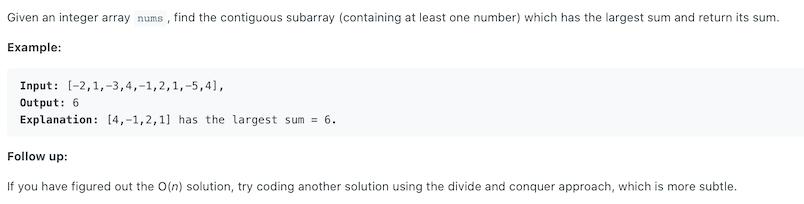
Find a contiguous subarray in an array that has the largest sum.
Similar Questions
- Easy - 121. Best Time to Buy and Sell Stock
- Medium - 152. Maximum Product Subarray
- Easy - 697. Degree of an Array
- Medium - 978. Longest Turbulent Subarray
Solution
Dynamic programming can be used here to tackle this problem.
We can use a one-dimensional array dp[i]
to represent the largest sum of subarray ending at index i
. In other words, the last element of this sub-array is the index i
element, and this subarray has the largest sum.
We have 2 situations here:
- If
dp[i-1] < 0
, thendp[i] = nums[i]
- If
dp[i-1] >= 0
, thendp[i] = dp[i-1] + nums[i]
Code:
1 | // dp array |
Time complexity O(n), and space complexity O(n).
Notice that we only used dp[i-1]
, so the whole dp array isn’t necessary here. Just need a variable to store the previous value.
1 | int previous = nums[0]; |
This way, time complexity is still O(n), but space complexity is optimized O(1).