Question
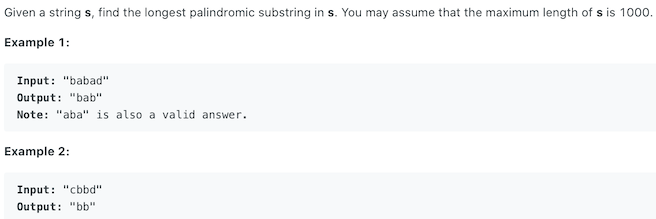
Dind the longest palindromic substring in a given string s
Similar Questions
- Hard - 214. Shortest Palindrome
- Hard - 336. Palindrome Pairs
- Medium - 516. Longest Palindromic Subsequence
- Medium - 647. Palindromic Substrings
Solution 1 - Brute Force
Enumerate all substrings, determine whether it is a palindrome string, and save the longest palindrome string.
Time complexity: need 2 for loop to enumerate all substring O(n2), and 1 for loop to determine if a string is palindrome. Thus overall time complexity O(n3).
Solution 2 - Expand from central
We know that the palindrome string must be symmetrical, so we can select a center each time, expand left and right, and determine whether the left and right characters are equal.
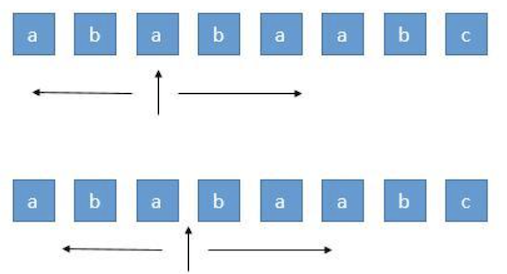
Because there are strings with odd length and even length, we need to expand from one central or from two central, so there are n + n-1 central in total.
1 | public String longestPalindrome(String s) { |
Time complexity: O(n2)
Solution 3 - Manacher’s Algorithm
Manacher’s Algorithm is a linear algorithm used to find the longest palindrome substring of a string. It was invented by a man named Manacher in 1975. The biggest contribution of this algorithm is to decrease the time complexity to Linear.
See: Manacher’s Algorithm. I will have a separate blog takling about this algothm in the future.