Question
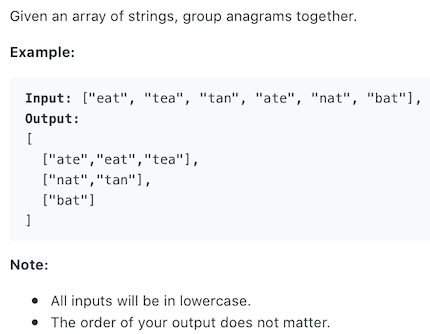
Given multiple strings, categorize them. As long as the strings contain exactly the same characters, they are counted as the same class, regardless of order.
Similar Questions
- Easy - 242. Valid Anagram
Solution
We can sort each string alphabetically, then we can map eat
, tea
, ate
to aet
. Others are similar.
1 | HashMap<String, List<String>> map = new HashMap<>(); |