Question
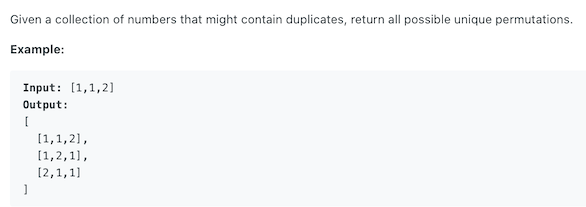
Similar to 46. Permutations, the difference is that there will be duplicates in the input, so using the previous algorithm will produce duplicate sequences. For example, [1 1]
, using the previous algorithm, the result must be [[1 1], [1 1]]
. In other words, a repeated sequence is generated. But we can modify the solution to the previous question to solve this problem.
Similar Questions
- Medium - 31. Next Permutation
- Medium - 46. Permutations
- Medium - 60. Permutation Sequence
- Hard - 996. Number of Squareful Arrays
Solution
Look at the previous solution:
1 | public List<List<Integer>> permute(int[] nums) { |
The first thing to resolve is these lines of code
1 | if (tempList.contains(num)) { |
There are no duplicate elements before, so we can directly determine whether there is a current element in tempList
and skip it if there is one. But here, because there are duplicate elements, this method is obviously not possible.
Put another way, we can use another list to save the index of the elements already in the current tempList
, and then decide if index already exist when adding new elements.
The second problem is that when we have duplicate elements, the generated result may contain same list, which is unnecessary.
The solution is to sort the array first, and then determine whether the previously added element is equal to the current element. If they are equal, skip it and continue to the next element.
Code:
1 | public List<List<Integer>> permuteUnique(int[] nums) { |
To solve the second problem !indexList.contains(i - 1)
is very important because the above indexList.contains(i)
code will make some elements skipped and not added to the tempList
, so we have to determine whether nums[i-1]
is the skipped element, if indexList.contains(i)
returns true, even if nums[i-1] == nums[i]
cannot skip the current element. Because the previous element nums[i-1]
was not added to the tempList
.