Question
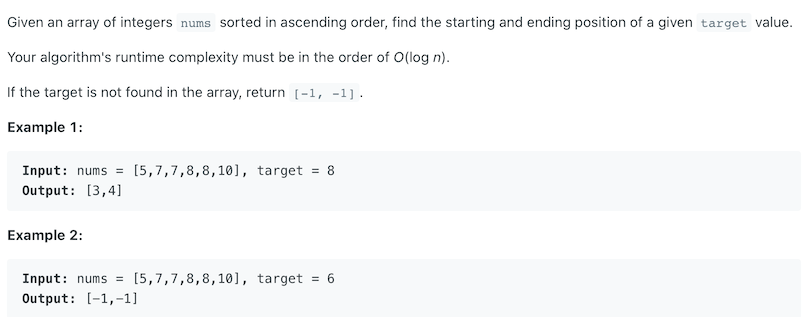
Find the first and last location of target value in a sorted array. Algorithm need to run in O(logn).
Similar Questions
- Easy - 278. First Bad Version
Solution 1 Linear Scan
Note that the array is sort. So we can make use of that. First scan from left to right. Once target value is found, stop scanning and record the index. Then scan from right to left, and record the index of first occurance of target value.
If target value is not found when scanning from left to right, then we can directly return [-1. -1]
as there is no target value in the array.
This algorithm is easy enough. However, time complexity is O(n), so it is not what we really want.
Solution 2 Binary Search
Solution referenced from here: https://leetcode.wang/leetCode-34-Find-First-and-Last-Position-of-Element-in-Sorted-Array.html
As we are targeting O(logn), binary search is a good candidate.
In a normal binary search, our algorith ends as soon as we find the target value. It may not be the leftmost target value. Like in the following case (pic taken from: ):
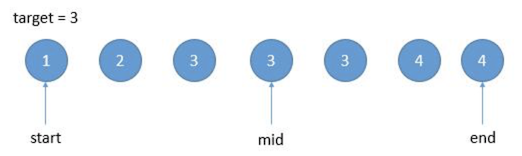
In this case we need to keep looking for the leftmost target value. So once we found the target value, insted of return, we make end = mid - 1
to keep searching. Eventually, the start
will stop at the leftmost target value.
We can do the same to find the rightmost target value. The end
will stop at the rightmost target value
Code:
1 | int start = 0; |
Time complexity O(logn), and space complexity O(1).