Question
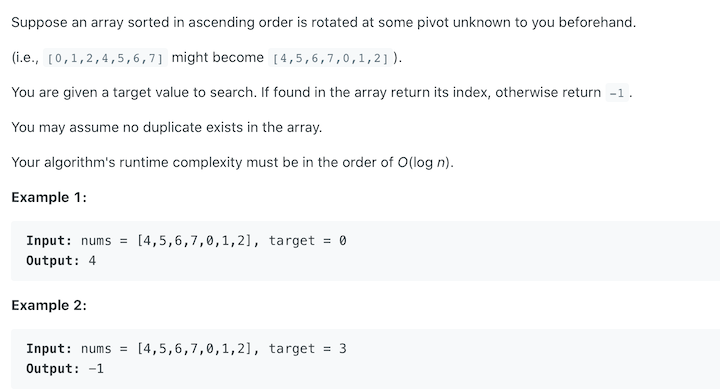
The original array is sorted, but rotated at some pivot index. We need to find the target value in the rotated array in O(logn). As we are looking for an O(logn) solution, we need to make use of binary search.
Similar Questions
Solution
Observe that for a given array, if we split it into half, at least one half is sorted. For example [4 5 6 7 8 1 2 3]
, split from 7
and get [4 5 6 7]
and [8 1 2 3]
. The first half is sorted.
Based on this observation, we can first find out which half is sorted (by comparing the end point values). Then check if target value is in this half. If it is, then discard the other half. If not, discard this half.
Code:
1 | int start = 0; |
Time complexity O(logn), and space complexity O(1).