Question
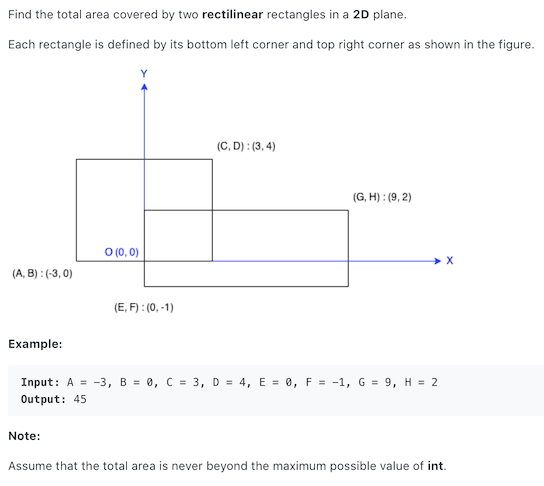
Find the area covered by the two rectangles.
Similar Questions
- Easy - 836. Rectangle Overlap
Solution
First, simplify the problem. Consider what if there are no overlapping areas?
Call the two rectangles A and B. There are four cases without overlapping. A is on the left of B, A is on the right of B, A is on the top of B, and A is on the bottom of B.
Call the two rectangles A
and B
. There are four cases without overlapping. A
is on the left of B
, A is on the right of B
, A
is on the top of B
, and A
is on the bottom of B
.
It is also very simple to determine the four situations above. For example, to determine whether A
is on the left of B
, you only need to determine whether the rightmost coordinate of A
is smaller than the leftmost coordinate of B
. Other situations are similar.
The area covered by the rectangle at this time is the sum of the areas of the two rectangles.
Next, consider the case of overlap.
At this time, we only require the area of the rectangle formed by the overlap, and then subtract the area of the overlapping rectangle from the area of the two rectangles.
It is also very simple to find the area of the overlapping rectangle. We only need to confirm the four sides of the overlapping rectangle.
For the left, just select the right one of the two rectangles’ left side.
For the top, just select the bottom one of the two rectangles’ top side.
For the right, just select the left one of the two rectangles’ right side.
For the bottom, just select the top one of the two rectangles’ bottom side.
Once determined, the area of the overlapping rectangles can also be calculated.
1 | int area1 = (C - A) * (D - B); |