Question
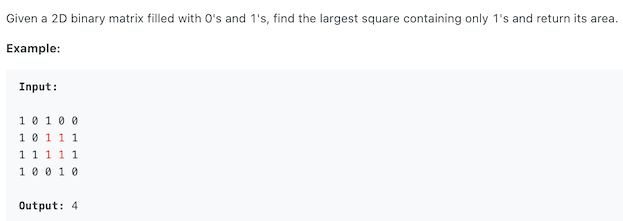
The output the maximum square area of 1 within a given array.
Similar Questions
- Hard - 85. Maximal Rectangle
- Medium - 764. Largest Plus Sign
Solution
Use dp[i][j]
to denote the maximum length of the square with matrix[i][j]
as the lower right corner. Then the recursion formula is as follows:
The initial condition is that
dp[i][j] = matrix[i][j]-'0'
in the first row and first column, which means thatdp[i][j]
is either0
or1
.Then there is recursion.
dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
That is, select the minimum value among the three points on the left, top, and upper left corner of the current point, and then add 1.
First of all, it must be clear that dp[i][j]
represents the maximum length of the square with matrix[i][j]
as the lower right corner.
Then we expand from the current point to the left and upward, we can refer to the figure below
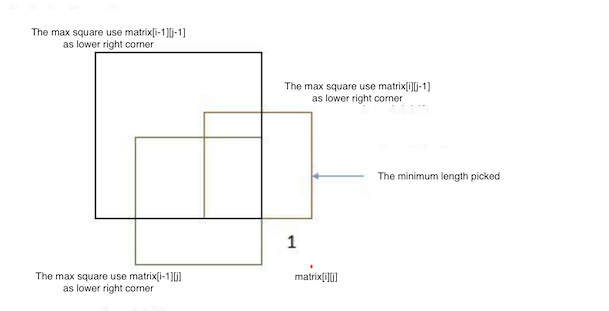
How much can we expand to the left? For dp[i][j-1]
and dp[i-1][j-1]
, select the smaller one to the left and upper left corner of the current point. That is, the largest square on its left and the largest square on its upper left corner, pick the smaller side length.
How much can we expand to up? dp[i-1][j]
and dp[i-1][j-1]
, choose the smaller one above the current point and the upper left corner. That is, the largest square on the upper side and the largest square on the upper left corner, pick the smaller side length.
Then choose the smaller one from expand the left and expand the up. Finally, add 1 to get the final side length.
Finally it is actually the smallest side length from the three squares.
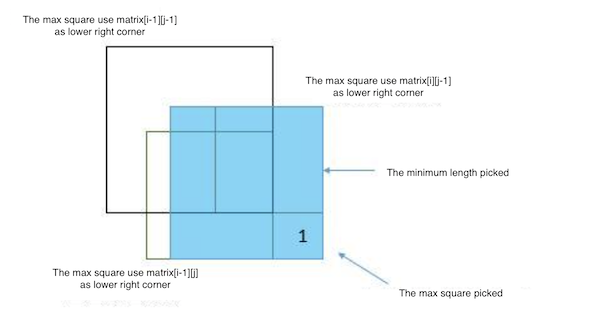
1 | public int maximalSquare(char[][] matrix) { |