Question
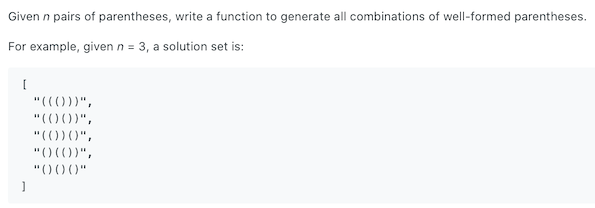
Give a number n
and return all valid parenthetical matches, exactly the opposite of 20. Valid Parentheses.
Similar Questions
- Medium - 17 Letter Combinations of a Phone Number
- Easy - 20. Valid Parentheses
Soultion - Brute Force
Enumerate all cases, each of which has left and right parenthesis, a total of 2n
digits, so a total of 22n cases.
1 | public List<String> generateParenthesis(int n) { |
Time complexity: O(n) is needed to judge whether each case is legal, so the time complexity is O(22nn)
Space complexity: O(22nn), multiply by n
because the length of each string is 2n
. In addition, this is assuming that all conditions are met, but it is not possible to meet all of them, and more accurate situations will be given later.
Solution
In previous solution, we keep adding left parentheses. In fact, if the left parenthesis exceeds n
, it is definitely not a legal case. Because the legal case must be n
left parentheses and n
right parentheses.
Another case is that if the total number of right parentheses is greater than the total number of left parentheses in the process of adding parentheses, no matter what is added later, it cannot be a legal case. Because each right parenthesis must match a previous left parenthesis, if there are more right parentheses than left parentheses, then there must be a right parenthesis that does not match the left parenthesis, no matter how many left parentheses are added later, it is useless. For example, n = 3
, there will be 6 parentheses in total. When we add ())
to 3 parentheses, there are 1 left parenthesis and 2 right parentheses. At this moment, the 3 parentheses behind it are doomed. Will not be a legal case anymore.
Based on the two points above, as long as we avoid them, we can guarantee that the brackets we generate must be legal.
1 | public List<String> generateParenthesis(int n) { |
The execution order of the recursion as follows:
1 | ( -> (( -> ((( -> ((() -> ((()) -> ((())) -> add to result |