Question
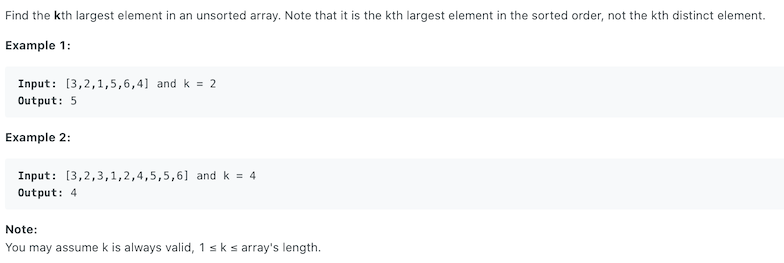
Find the kth largest number in an array.
Similar Questions
- Medium - 324. Wiggle Sort II
- Medium - 347. Top K Frequent Elements
- Easy - 414. Third Maximum Number
- Easy - 703. Kth Largest Element in a Stream
- Medium - 973. K Closest Points to Origin
Solution - Brute Force
Use quick sort to sort from large to small, and return the kth number.
We can directly use the sorting algorithm provided by java, and because the default is to sort from small to large, so we can return the last kth number.
Solution - Priority Queue
We can use the priority queue to build a maximum heap, and then pop the elements in turn. The kth element that is popped is what we are looking for.
Here we can use the PriorityQueue
provided by java.
Java default is to build the minimum heap, so we need a Comparator
to change the priority.
Introduction to PriorityQueue
can be found here: Priority Queue
1 | public int findKthLargest(int[] nums, int k) { |