Question
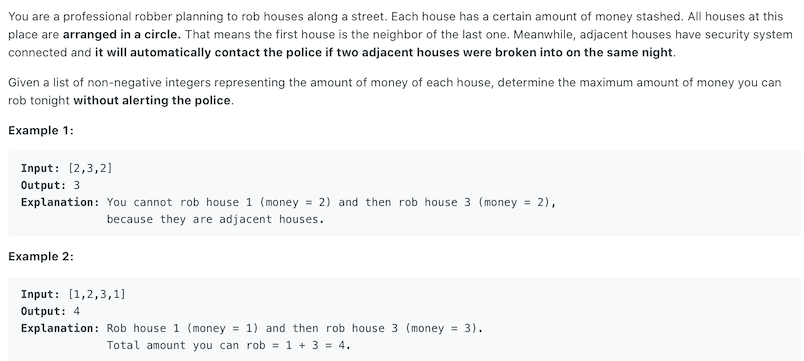
Gavin an array, each element represents the deposit of the store. A thief went to steal the store at night. Need to determine how much money can be stolen at most. The neighboring shops cannot be stolen otherwise the alarm will sound. The stores are distributed in a circle, so the first and last ones are also counted as adjacent stores.
Similar Questions
- Easy - 198. House Robber
- Medium - 337. House Robber III
- Hard - 600. Non-negative Integers without Consecutive Ones
Solution
Because first and last one can’t be stolen together, so we have 2 situations:
- Stealing the first one. We need to find the maximum money of stealing in the first
n - 1
households. That is, not considering the maximum gain of the last one. - Do not steal the first one. We need to find the maximum money from the second to the last one. That is, do not consider the maximum gain of the first one.
Then we only need to return to the larger of the above.
1 | public int rob(int[] nums) { |