Question
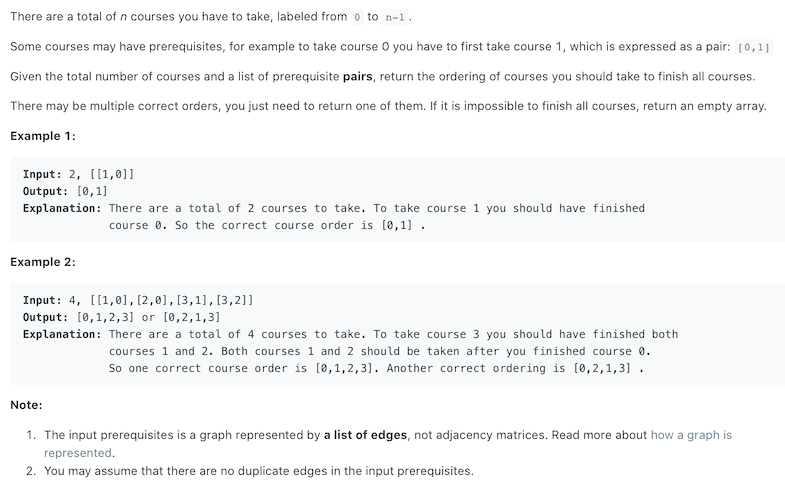
A extension on 207. Course Schedule. Given the relationship of n
groups of prerequisite courses, [m, n]
means that you must take n
courses before taking m
courses. Output a lesson sequence.
Similar Questions
- Medium - 207. Course Schedule
- Medium - 310. Minimum Height Trees
- Medium - 630. Course Schedule III
Solution
207. Course Schedule is to determine whether there is a sequence to finish all the lessons. Here we need to output the sequence. The idea is basically the same.All relationships can be regarded as the edges of the graph, and all the edges constitute a directed graph.
For [[1,3], [1,4], [2,4], [3,5], [3,6], [4,6]]
, it can be seen as the figure below, the arrow points to the prerequisite class.
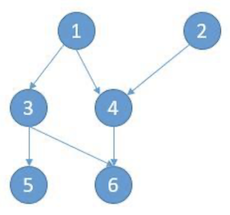
If we want to finish all the classes, there must be some classes that have no prerequisite, such as 5
and 6
in the picture above. Then we can delete the 5
and 6
nodes.
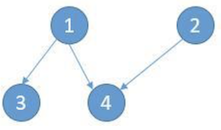
Then we can take 3
and 4
, and 3
and 4
are deleted for the same reason.
Then we can take 1
and 2
. Therefore, all classes can be completed.
We can use the outDegree
to record the number of prerequisite courses for all nodes. When deleting a node, the number of prerequisite courses of the corresponding node can be reduced by one.
In the end, it is only necessary to determine whether the number of prerequisite courses of all nodes is all 0
.
Just need to modify the code of 207. Course Schedule to record the element that is popped from the queue.
1 | public int[] findOrder(int numCourses, int[][] prerequisites) { |