Question
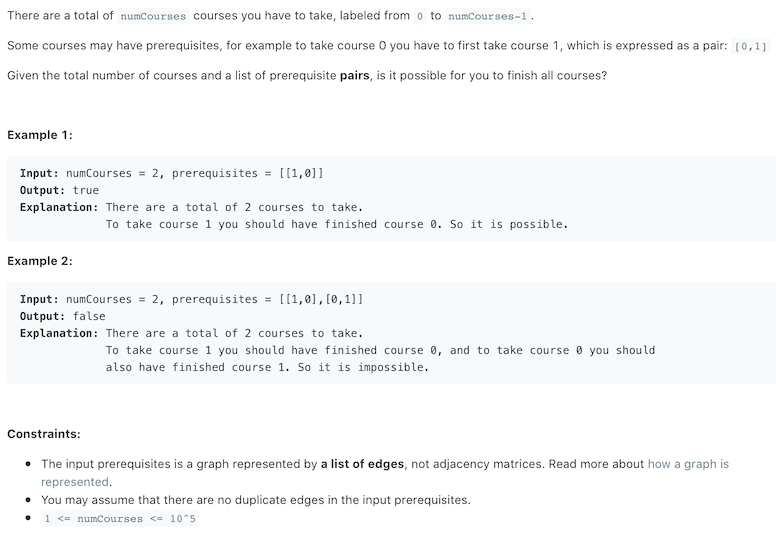
Given the relationship of n
groups of prerequisite courses, [m, n]
means that n
class must be taken before m
class. Output whether a student can successfully complete all classes.
Similar Questions
- Medium - 210. Course Schedule II
- Medium - 310. Minimum Height Trees
- Medium - 630. Course Schedule III
Solution
All relationships can be regarded as the edges of the graph, and all the edges constitute a directed graph.
For [[1,3], [1,4], [2,4], [3,5], [3,6], [4,6]]
, it can be seen as the figure below, the arrow points to the prerequisite class.
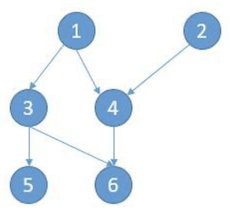
If we want to finish all the classes, there must be some classes that have no prerequisite, such as 5
and 6
in the picture above. Then we can delete the 5
and 6
nodes.
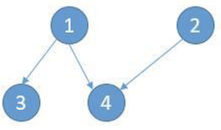
Then we can take 3
and 4
, and 3
and 4
are deleted for the same reason.
Then we can take 1
and 2
. Therefore, all classes can be completed.
In terms of codes, the graph is represented by an adjacency list. In addition, we don’t need to actually delete the node, we can use the outNum
variable to record the number of prerequisite courses for all nodes. When deleting a node, the number of prerequisite courses of the corresponding node can be reduced by one.
Lastly, we only need to determine the number of prerequisite courses of all nodes is 0
.
1 | public static boolean canFinish(int numCourses, int[][] prerequisites) { |