Question
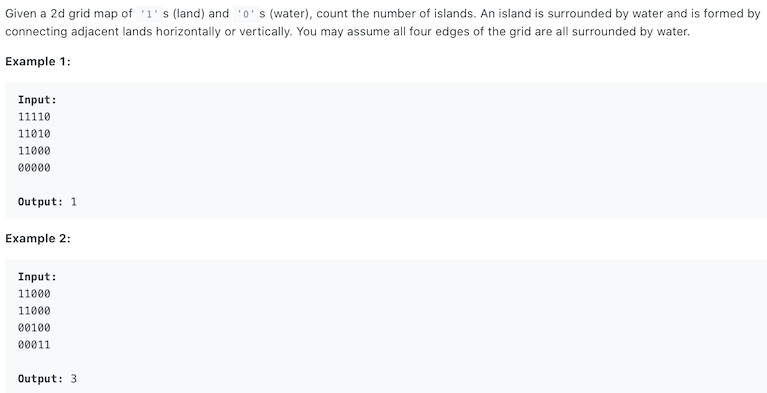
Given a two-dimensional array, 1
as the land and 0
as the sea, connecting the land to form an island. Think of the area outside the array as the sea, and determine how many islands there are.
Similar Questions
- Medium - 130. Surrounded Regions
- Medium - 695. Max Area of Island
Solution
The idea is very simple, we only need to traverse the two-dimensional array. When we meet 1
, just mark the current 1
and all the 1
s connected to it as 2. Then record how many 1
we have met, which means there are several islands. See the example below.
1 | [1] 1 0 0 0 |
Another problem is how to mark all connected 1
s. It is also very straightforward. We can directly do a DFS using recursion.
1 | public int numIslands(char[][] grid) { |