Question
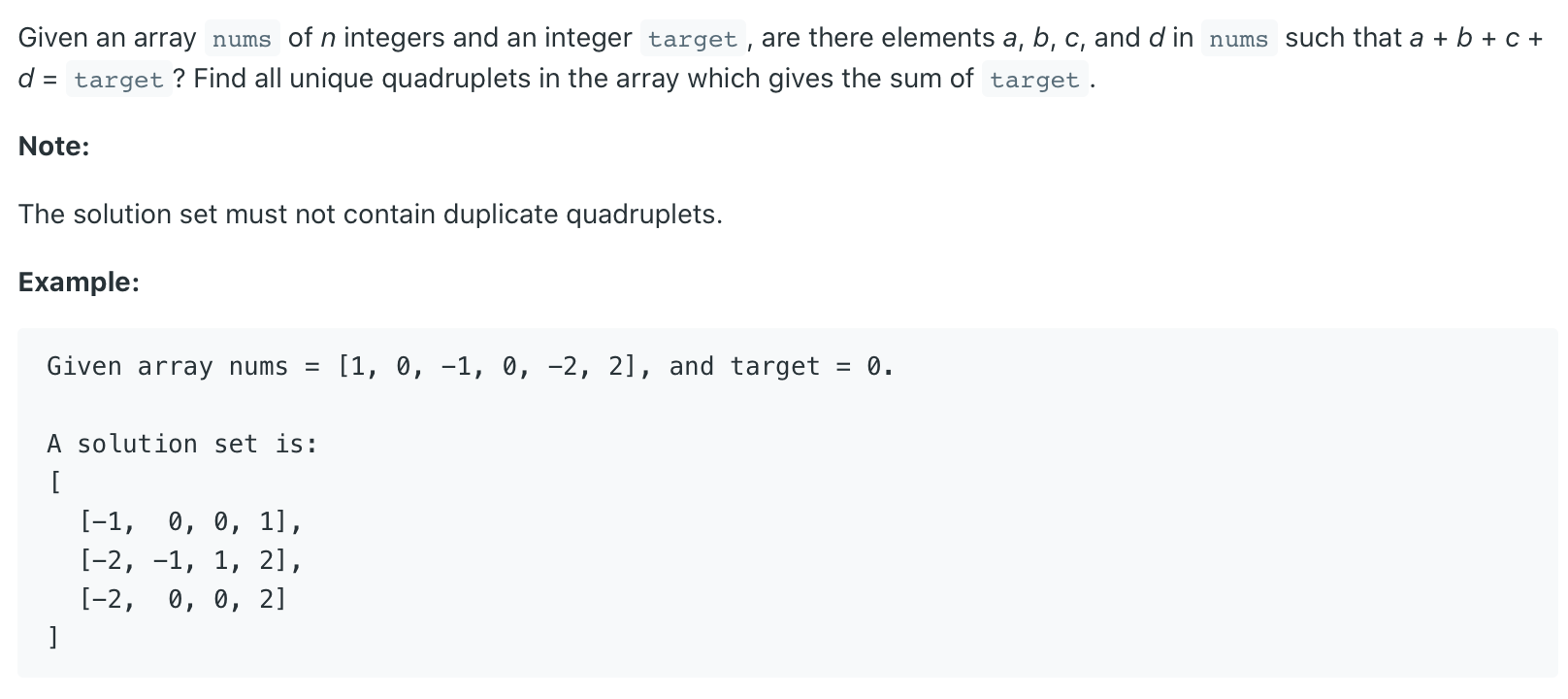
Similar to Question 15: 3Sum. This time we want to find all 4 numbers that add up to the target value.
Similar Questions
- Easy - 1. Two Sum
- Medium - 15: 3Sum
- Medium - 454. 4Sum II
Soultion
The idea is excatly same with Question 15: 3Sum. Just adding another layer of for loop.
- Sort the array
- First for loop to get the first number. (0 - num.length - 3)
- Use an If statement to avoid duplicate. (Skip the same number)
- Second for loop to get second number. (i + 1 to num.length - 2)
- Another If statement to avoid duplicate. (Skip the same number)
- Now we use 2 pointers
head
andtail
to find remaining 2 numbers sum = target - num[i] - num[j]
- Depending on the comparison of sum and target:
8.1 sum = target: add to the result list, and move inwardhead
andtail
until all same number are skipped
8.2 sum < target:head++
8.3 sum > target:tail--
1 | List<List<Integer>> results = new ArrayList<>(); |
Time complexity O(n3), and space complexity O(1).