Question
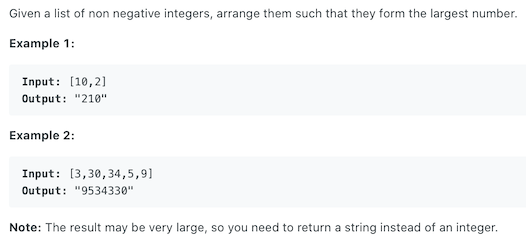
Given an array, arrange the numbers arbitrarily to form a number with the largest value.
Solution
Intuitively, we should choose the number with largest highest number first. For numbers that start with 9, choose 9 first, then choose numbers that start with 8, then choose ones that start with 7 … and so on.
For example, for 5
, 914
, 67
, choose 914
first, then 67
, and then 5
, to form 914675
. We try to select the number with largest highest number each time to ensure the final number be the biggest.
The next question is what if the highest digit is the same?
- If two numbers are equal in length, such as
34
and30
, then obviously, choose the larger one. - If two numbers are not equal in length, such as
3
and30
, then choose3
or30
first?
We only need to contac them together and then compare. That is, compare 330
and 303
, it is obviously 330
big, so we choose 3
first.
If we think about it, we can find that all we need to do is sort the array by our rules and contac it afterwards.
For the comparision rule, all we have to do is compare the combined number and determine which one should comes first.
For example, for 93
, 234
, we will compare 93234
and 23493
. Then we will choose 93
.
1 | public String largestNumber(int[] nums) { |