Question
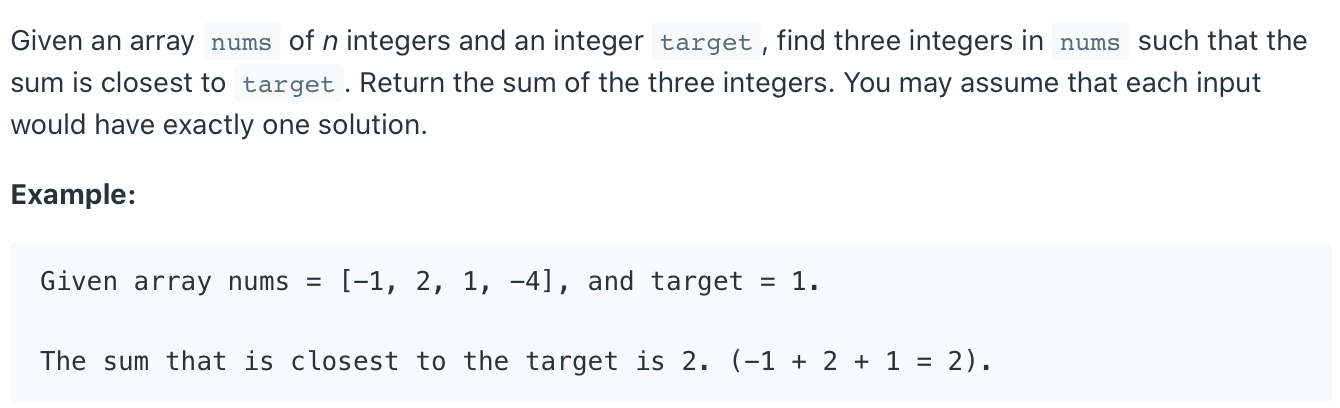
Similar to Question 15: 3Sum. This time we want to find the 3 sum that is closest to the target value.
Similar Questions
- Medium - 15: 3Sum
Solution 1
Brute force. We need 3 nested loop to get all possible sums, and then get the sum that is closest to the target. Time complexity is O(n3). Space complexity O(1).
Solution 2
We can make use of the similar idea of Question 15: 3Sum.
- Sort the array
- Loop the array to get the first number
nums[i]
- Use 2 pointers
head
andtail
to loop the remaining array (head = i + 1
) sum = nums[i] + nums[head] + nums[tail]
- Depending on the comparison of sum and target:
5.1 sum = target: then we can return the value as it as closest to the target
5.2 sum < target:head++
5.3 sum > target:tail--
- Use a variable to keep track of the smallest distance
Code:
1 | // keep trakc of minimun distance |
We are running 2 loops (O(n2)), and space complexity O(1).