Question
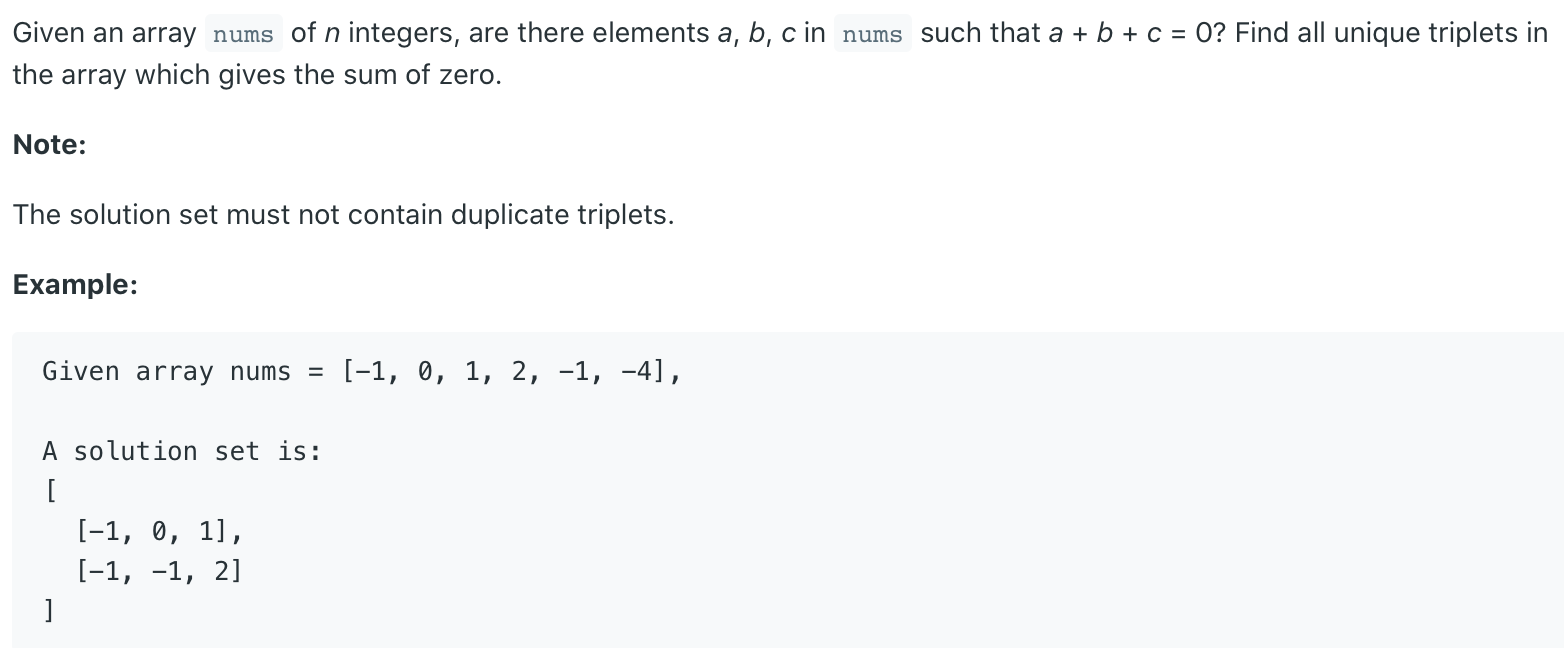
Given an array, find all unique triplets that add up to 0
Similar Questions
- Easy - 1. Two Sum
- Medium - 16: 3Sum Closest
- Medium - 18: 4Sum
Solution 1
Brute force solution. We need 3 nested for loops. If we found that nums[i] + nums[j] + nums[k] == target
, then need to check if the solution already exist (which takes O(n)). So the overall complexity is O(n3). Space complexity O(1).
Solution 2
Reference https://leetcode.com/problems/3sum/discuss/7380/Concise-O(N2) as a O(n2) solution.
The main idea is to iterate through the array, fix one number as sum, and find another 2 numbers that add up to -sum. The clever thing is how to find the other two numbers in O(n).
The achieve that, first we need to sort the array.
Then we get num[i]
as target , and try to find if there are 2 element add up to -num[i]
. As the array is sorted, we can use 2 pointers (low and high) at head and tail, so find 2 elements takes O(n).
num[low] + num[high] > target
, high = high -1. As we are greater than target, we need to decrease the high pointer to make sum smaller.num[low] + num[high] < target
, low++. As we are smaller than target, we need to increase the low pointer to make sum larger.
To avoid add duplicate result, we need to move the pointer to point to different number with num[low]
and num[high]
. For example, let’s say the array is [-2, -1, 0, 0, 1, 1, 2, 3]
. The num[i] = -2
. We found that num[1] + num[5] + num[i] = 0
. To continue the loop, we need to move high = 5
to high = 3
. If we don’t have this step, then when the loop continues, we will have num[1] + num[4] + num[i] = 0
and this result is duplicate with the result we already have.
Code:
1 | List<List<Integer>> results = new ArrayList<>(); |