Question
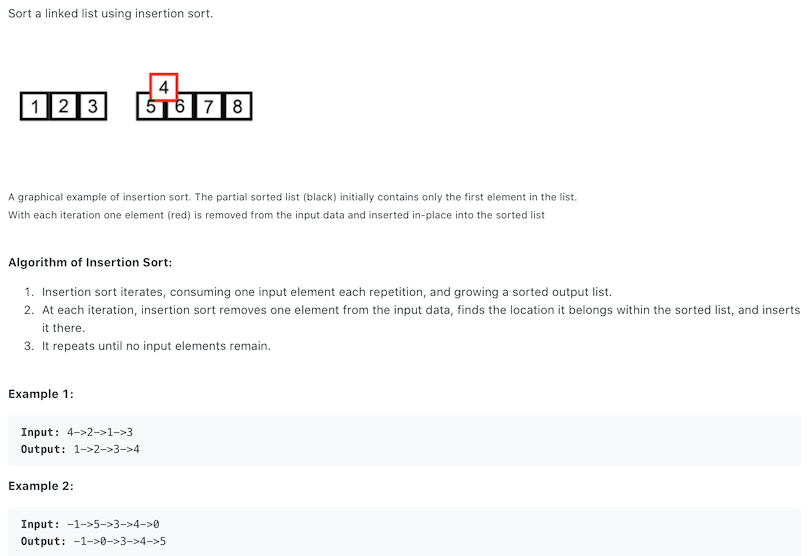
Implement insertion sorting based on linked lists.
Similar Questions
- Medium - 148. Sort List
Solution
The so-called insertion sorting is to take one number at a time and insert it to the correct position.
For exampel:
1 | 4 2 1 3 |
To implement it, because we want to take out an element from the linked list that we want to insert it into the sorted list, we must first traverse the sorted list, find the first position that is larger than the element to be inserted, and insert the element in front.
As for insertion, we need to know the node before the insertion position, so we can use node.next
to compare with the node to be inserted, node
is the node before the insertion position.
The head pointer is already at the forefront, so we can use a dummy pointer to unify the situation of the head pointer.
1 | public ListNode insertionSortList(ListNode head) { |