Question
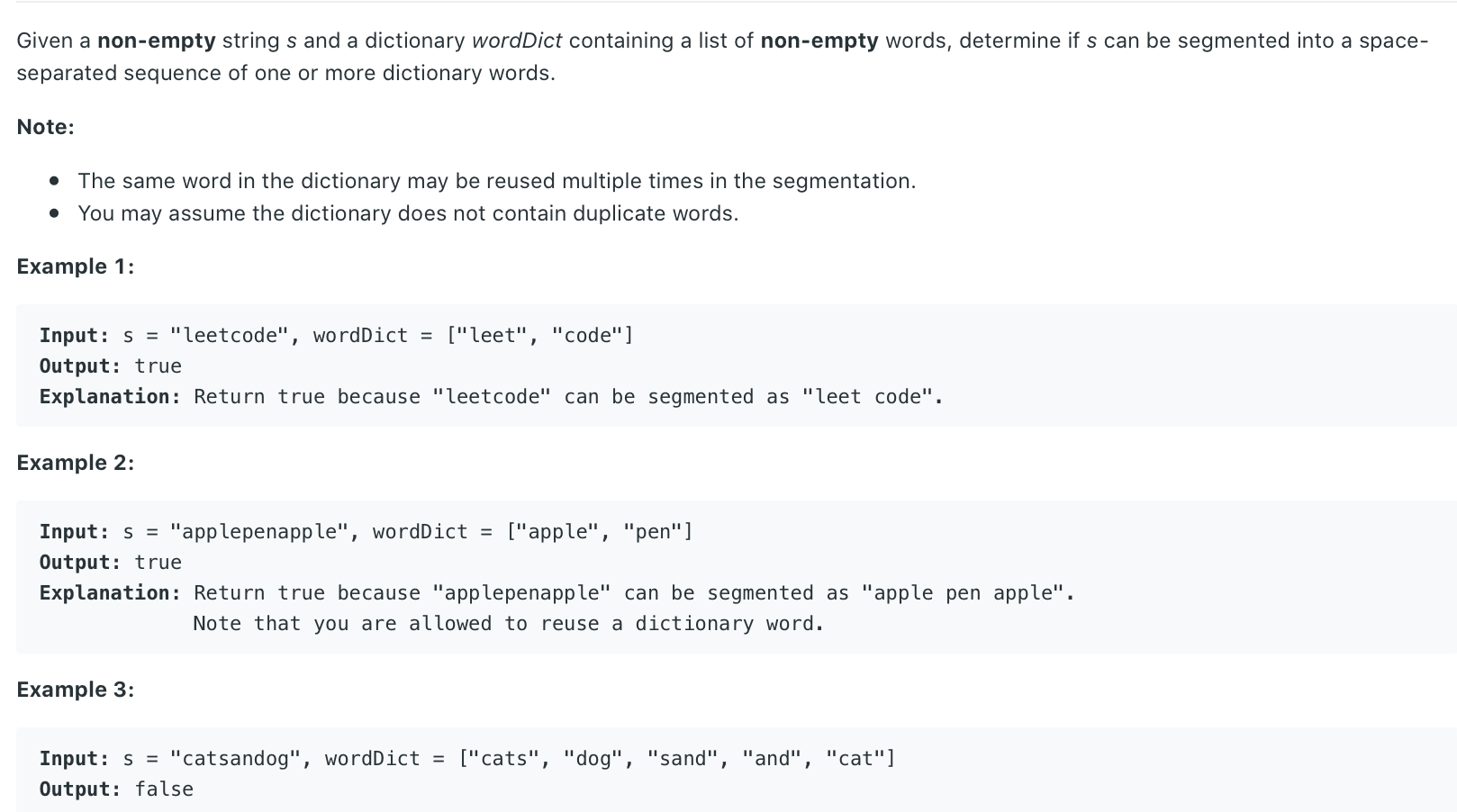
Give a string, and some words, and determine if the string can be composed of these words. Each word can be used multiple times or not.
Similar Questions
- Hard - 140. Word Break II
Solution
Divide and conquer, big problems are converted into small problems and solved by small problems.
We now need to determine whether the target string s
can be composed of wordDict
.
Use canBreak[i]
to indicate whether the substring s[0, i)
can be composed of wordDict
.
Suppose we know canBreak[1]
, canBreak[2]
, canBreak[2]
, … canBreak[len - 1]
, that is, whether all substrings except s
can be composed of wordDict
.
Then we can know
1 | canBreak[len] = canBreak[1] && wordDict.contains(s[1,len)) |
canBreak[len]
represents whether s
can be composed of wordDict
.
1 | public boolean wordBreak(String s, List<String> wordDict) { |