Question
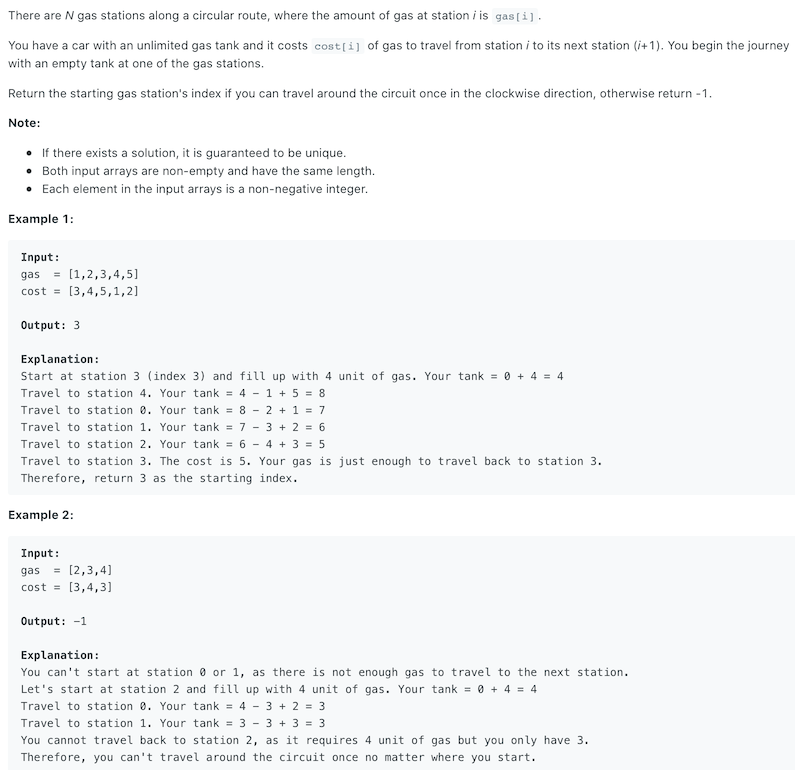
Just understand this question as the picture below:
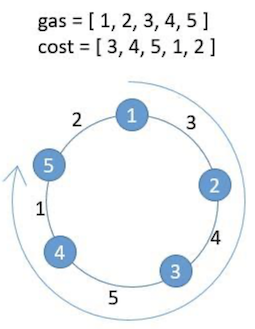
Each node represents the amount of oil added, and each edge represents the amount of oil consumed. The meaning of the question is to ask us which node we start from and return to that node. We can only go clockwise.
Solution
For this question, we can just use Brute Force.
Consider whether starting from the 0
point, and can return to the 0
point.
Consider whether starting from the 1
point, and can return to the 1
point.
Consider whether starting from the 2
point, and can return to the 2
point.
…
Consider whether starting from the n
point, and can return to the n
point.
Since it is a circle, we need to take the remainder when we get the next point.
1 | // already assume gas and cost are non-empty and have same length |