Question
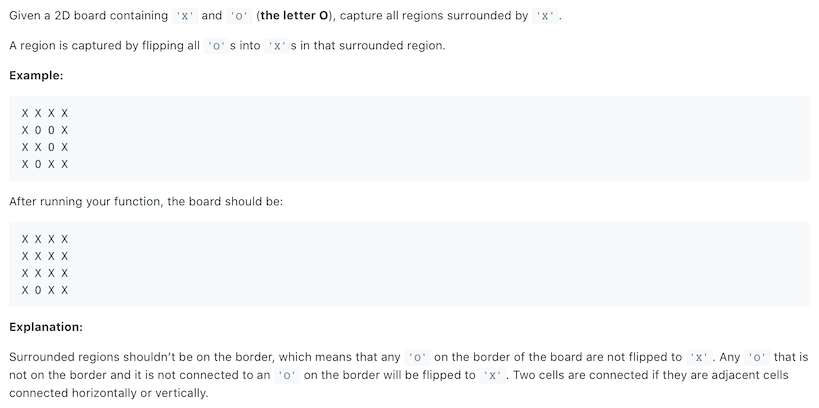
It’s a little bit like Go, turning the O
enclosed by X
into X
, and the O
at the boundary will not be enclosed. If O
and O
on the boundary are connected, then these O
are counted as unenclosed, as in the example below.
1 | X X X X X |
The example above only needs to change one O
.
1 | X X X X X |
Similar Question
- Medium - 200. Number of Islands
Solution - DFS
Consider adjacent O
as a connected graph, and then start DFS from each O
.
If O
does not reach the boundary after the traversal is completed, we change the current O
to X
(meaning this O
is not connected to a O
at the boundary, hence enclosed by X
).
If the boundary O
is reached during the traversal, DFS is ended directly, and the current O
does not need to be changed (because it’s connected to a O
at the boundary).
Then continue to consider the next O
, continue to do DFS.
Solution - Union Find
Union find data structure: https://en.wikipedia.org/wiki/Disjoint-set_data_structure
In computer science, union find is a tree-shaped data structure, used to deal with some disjoint sets merge and query problems. There is a union-find algorithm that defines two operations for this data structure:
- Find: Determine which subset the element belongs to. It can be used to determine whether two elements belong to the same subset.
- Union: Merging two sub-collections into the same set.
In order to define these methods more precisely, we need to define how to represent collections. A common strategy is to select a fixed element for each collection, called a representative, to represent the entire collection. Next, find(x)
returns the representatives of the set to which x
belongs, and union()
uses the representatives of the two sets as parameters.
Knowing the union-find, it’s easy to find a solution to the problem. We can find that what we do is the problem of classification. In fact, O
is ultimately divided into two categories, one can be connected to the boundary, and the other can not be connected to the boundary.
We need to iterate over each O
node and merge it with the O
nodes above, below, left and right.
If the current node is the boundary O
, merge it with the dummy node (a node outside all nodes). Finally, all O
nodes and dummy nodes connected to the boundary will be combined into one category. The other O
nodes that are not connected to boundary O
are another category.
Finally, we only need to find out whether each O
node is the same as the dummy node.
1 | public void solve(char[][] board) { |
1 | public class UnionFind { |