Question
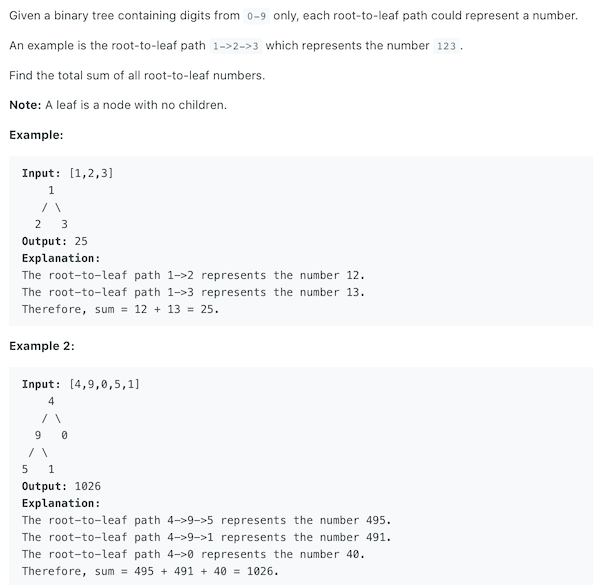
Given a binary tree, the path from the root node to the leaf node forms a number. Calculates the sum of all numbers.
Similar Question
- Easy - 112. Path Sum
- Hard - 124. Binary Tree Maximum Path Sum
- Medium - 988. Smallest String Starting From Leaf
Solution
We need to traverse the binary tree, and record the sum of the current path during the traversal process. When it comes to traversal, it is nothing more than BFS
and DFS
. If BFS
is performed, we need to maintain the sum of multiple paths during the process, so we choose DFS
.
When it comes to DFS
, we can use recursion, or use the stack.
When it comes to recursion, we can use both backtracking and divide and conquer.
Let’s look at the backtracking solution.
The idea of backtracking is to keep traversing in depth until a solution is obtained and record the current solution. Then return to the previous state to continue the depth traversal.
So we need to define a function to get this solution.
1 | private void dfs(TreeNode node, int tempSum) |
This function means that when traversing from the root
node to the node
, the cumulative sum of the paths is cursum
.
Here we use a global variable result
to store the sum of each path.
So the exit of backtracking is that when we reach the leaf node, save the current accumulated path sum.
1 | if (node.left == null && node.right == null) { |
Then just try the left and right subtrees separately.
1 | int result = 0; |