Question
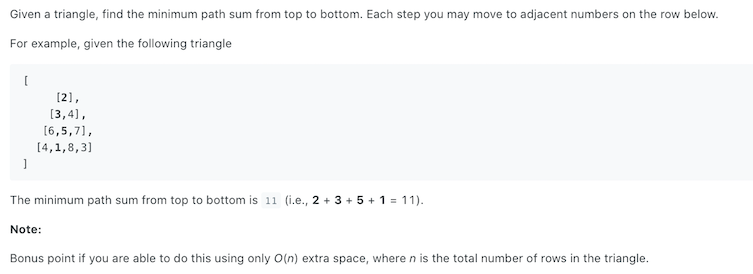
From current layer can only choose two adjacent elements to walk down. For example, the third layer of 5
, we can only choose the fourth layer of 1
and 8
, starting from the top. Take a path to the bottom with the smallest sum.
Solution
We can construct the result from top to down, get the possible minimum at a specific positon. Lastly the minimum in the last is the answer we want to get.
There are two options to get to the current position. Choose a smaller one and add the number at the current position.
The point to note is that the left and right borders need to be considered separately, because there is only one position to reach the left and right borders.
We are updating layer by layer, and updating the current layer only requires the information of the previous layer, so we do not need a two-dimensional array, only a one-dimensional array.
E.g
1 | [2], |
1 | public int minimumTotal(List<List<Integer>> triangle) { |