Question
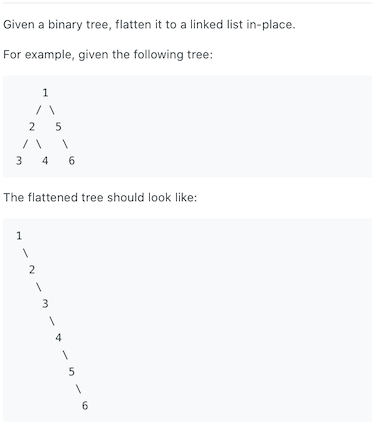
Expand a binary tree into a linked list.
Similar Question
Solution
It can be found that the order of flatten is actually the pre-order traversal of the binary tree. So the problem is actually to convert the binary tree through the right pointer to form a linked list.
E.g. given:
1 | 1 |
Try to get:
1 | 1 -> 2 -> 3 -> 4 -> 5 -> 6 |
Once we have this pre-order list, we can tranverse through it. Each time a node is traversed, the right pointer of the previous node is updated to the current node.
The pre-order list is 1 2 3 4 5 6
.
Traverse to 2
and point the right pointer of 1
to 2
. 1-> 2
3 4 5 6
.
Traverse to 3
and point the right pointer of 2
to 3
. 1-> 2-> 3
4 5 6
.
…
Because we saved the right child with the list, there is no need to worry about the right child being lost.
Code:
1 | public void flatten(TreeNode root) { |