Question
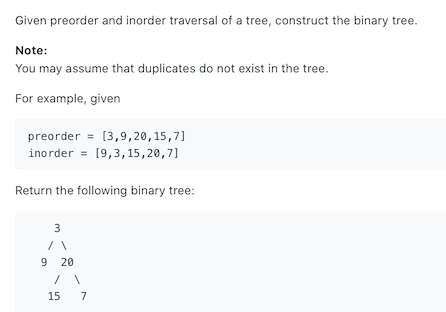
Restored binary tree according to the pre-order and in-order traversal.
Similar Question
Solution
The order of the pre-order traversal
is the root node, the left subtree, and the right subtree. The order of the in-order
traversal is the left subtree, the root node, and the right subtree.
So we just need to get the root node according to the pre-order traversal, and then find the position of the root node in the in-order traversal. The left side is the nodes of the left subtree, and the right is the nodes of the right subtree.
The left and right subtrees can then be generated recursively.
For example
1 | preorder = [3,9,20,15,7] |
In fact, we don’t need to really split the pre-order and in-order, we just need to use two pointers to point to the beginning and end respectively. Note that the range of the array pointed to by the two pointers below includes the left boundary, not includes the right boundary.
1 | public static TreeNode buildTree(int[] preorder, int[] inorder) { |