This blog will introduce the join()
method in Thread
. The content includes:
- Introduction to
join()
join()
source code analysis (based on JDK11.0.5)- Example
Introduction to join()
join()
is defined in Thread.java
.
join()
will let the main thread
wait for the end of the sub-thread
to continue running. Look at the examples:
1 | // Main thread |
Son thread
is created and started in Father thread
, so Father thread
is the main thread and Son thread
is the sub-thread.
The Father thread
create a new sub-threads through new Son()
, and then start sub thread s.start()
and call s.join()
. After calling s.join()
, the Father thread
will wait until the sub thread has finished running. After the sub thread s
has finished running, the Father thread
can continue to run. This is what we mean by join()
, so that the main thread will wait for the child thread to finish before continuing to run!
join() source code analysis (based on JDK11.0.5)
1 | public final void join() throws InterruptedException { |
From the code, we can find out that when millis == 0
, it will enter while(isAlive ())
loop. That is, as long as the child thread is alive, the main thread will keep waiting.
Let look back at the above example:
Although s.join()
is called in the Father thread
, s.join()
is the method call on the sub thread s
. Then, isAlive()
in the join()
method should determine whether the sub thread s
is in the Alive state. The corresponding wait(0)
should also let the sub thread s
wait. But if this is the case, how could s.join()
make main thread
wait until the sub thread s
is completed? It should instead make the sub thread s
wait (because the wait method of the subb thread s
is called)?
Well, the answer is that wait()
will make the current thread wait, and the current thread here refers to the thread currently running on the CPU
. Therefore, although the wait()
of the sub thread
is called, it is called through the main thread
. Therefore, it is the main thread
that waits, not the sub thread
!
Example
After understanding the function of join()
, let’s look at the usage of join()
through examples.
1 | public class JoinDemo { |
Results:
1 | t1 start |
The execution sequence is shown in the figure:
- Create a new
thread t1
in themain thread
withnew ThreadA("t1")
. Next, startthread t1
witht1.start()
and executet1.join()
. - After executing
t1.join()
, themain thread
will enter theblocked state
and wait fort1
to finish running. Aftert1
ends, it will wake up themain thread
, andmain thread
regains the cpu execution right and continues to run.
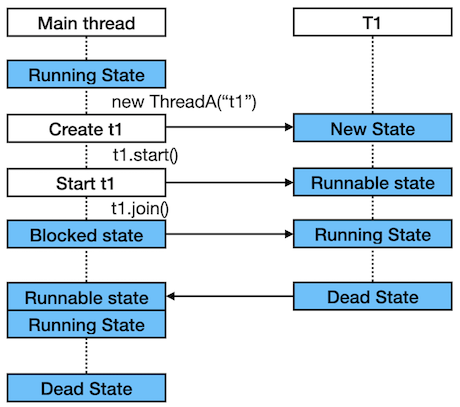